VCE Software Development Units 3 & 4
PROGRAMMING YOUR SAT
​
A major part of Software Development is the plannng and development of your own software solution in a programming language. At our school we program in Visual Basic so I have compiled some freely available onlie tutorials below as well as examples of key control structures that are required for your SAT. Ensure you are familiar with VCAAs Software Development Programming Requirements (2018) and you are working with the 2018 SAT Criteria.
​
​
INTRODUCTIONS TO CODING IN VISUAL BASIC
There are plenty of online resources that allow you work through Visual Basic exercises step by step:
​
Check out Passy's World of ICT Videos Designed by an IT Teacher
​
​
Here is a series of EXCELLENT VIDEOS that take you through Visual Basic 2017.
​
This is a five part video that takes you through creating a database using Visual Basic
​
SAVING AND RETRIEVING DATA FROM EXTERNAL FILES
There are some excellent videos online to connect your VB solution to an Access Database. This series of videos takes you through the development of a full project from start to finish.
​
Firchild has everything your need!
​
VB/Access Video 7.1 Overview of Project
VB/Access Video 7.2 Set up Tables in Database
VB/Access Video 7.3 Setting Queries
VB/Access Video 7.4 Connecting your database
VB/Access Video 7.5 Data Adapter
VB/Access Video 7.6 Navigating Records
VB/Access Video 7.7 Updating Records
VB/Access Video 7.8 Adding new records
VB/Access Video 7.9 Deleting records
VB/Access Video 7.10 Saving Data & Validation
VB/Access Video 7.11 Passing a Query to dataset
VB/Access Video 7.12 Passing DataSet to a DataGrid
VB/Access Video 7.14 Using a Query to Calculate
VB/Access Video 7.15 Validation to stop double bookings
VB/Access Video 7.16 Create a Main Console
VB/Access Video 7.17 Complete login
​
Interface
Programming requirements for interface layer:
-
develop a graphical user interface (GUI), for use in digital systems such as laptops, gaming consoles, mobile phones, tablets, robots.
-
Note that databases are not to be used in the interface layer.
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
In the simple Curtain Calculator above you can see that the interface is contained in the VB form using GUI objects. There maybe a Access Database storing the data, or perhaps an XML file, but the user does not interact with them directly.
​
Logic
Programming requirements for logic layer:
-
construct and use data structures
​
You will need to use data structure in your solution. These include RECORDS, 1D ARRAYS, ASSOCIATIVE ARRAYS and HASH TABLES.
​
A record can be stored in an XML file which can be accessed by VB. the PDF docment here shows you how to:
-
create an XML file withing Visual Basic
-
read data into Visual Basic from an XML file
-
use basic text files to store and retrieve data
​
A one dimentional array can be declared within the code or read in from an external file. Her is an example of a ID Array
in Visual Basic.
​
'Declares the array
Dim Names(5) as String
​
Names(0) = Bill
Names(1) = Betty
Names(2) = Brian
Names(3) = Belinda
Names(4) = Betina
​
'This will display the first name in the lable object
lblfirstName.Text = Name(0)
​
'This will read in a name and put a new name into the first elelment of the array
Names(0) = txtName.Text
​
​
An Associative Array (or Dictionary) is an array that contains more than one column. An example in Visual Basic is below.
​
​
​
'Below we set the contents of the first two columns of the Array I have put spaces either side so as to make it easier to read.
Team(0, 0) = " 1 "
Team(0, 1) = " Goal Keeper "
Team(1, 0) = "2 "
Team(1, 1) = " Right Full Back "
Team(2, 0) = "3 "
Team(2, 1) = " Left Full Back "
Team(3, 0) = "4 "
Team(3, 1) = " Centre Half Back "
Team(4, 0) = "5 "
Team(4, 1) = " Centre Half Back "
Team(5, 0) = "6 "
Team(5, 1) = " Defensive Midfielder "
Team(6, 0) = "7 "
Team(6, 1) = " Right Winger "
Team(7, 0) = "8 "
Team(7, 1) = " Central Mid Fielder "
Team(8, 0) = "9 "
Team(8, 1) = " Striker "
Team(9, 0) = "10 "
Team(9, 1) = " Attacking Midfielder "
Team(10, 0) = "11 "
Team(10, 1) = " Left Winger "
​
Hash Tables are complex solutions that use a calculation with a prime number to find a unique location (key) in an array to make it easier to find when you have a large file of unsorted data.
​
In a basic address book, you might have:
-
Bill
-
Surpreet
-
Jane
-
Nqube
-
Quentin
The problem with locating these names in an address book alphabetically is that is leaves spaces in the table between Bill and Michael empty wasting space. Also a linear search s\would still be required under each alphabetical section.
​
Hash tables provide a unique identifyer based on the content of the data. If we uses a basic converst\ion of a=1, b=2, c=3 etc we could convert "Jane" to:
(J)11 + (a)1 + (n)15 + (e)5 = 32
​
Unfortunately if "Neaj" is added, his converted number would also be 32. So we use a Hash Function that allocates values depending on the location of each character in the string. We use a prime number which helps use create unique values (in this example we will use 7).
Hash(Current Letter) = (Prime Number(7) * Hash from previous value) + value of current letter.
Example:
JANE
​
Hash = 0
Hash (J) = (7 * 0) + 11 = 11
Hash (A) = (7 * 11) + 1= 78
Hash (N) = (7 * 78) + 15 = 561
Hash (E) = (7 * 561) + 5 = 3,932
​
Hash (JANE) = 3,932
​
To provide more details of VB examples - see the searching techniques below. Here is a VB tutorial is setting up a Hash Table
Try this site for more information.https://www.dotnetperls.com/hashtable-vbnet
​
​
​
​
​
​
​
​
​
​
Logic
Programming requirements for logic layer:
-
design and apply data validation techniques
​
Validation is essential to an effective and efficient solftware solution.. This process ensures all data input into the system is checked and responded to appropriately. The user needs to be provied feedback if they enter data that will be processed by the solution.
​
EXISTENCE CHECKING
​
If the user needs to enter data into a field and they leave it blank or unselected, the user needs to be prompted to enter their data before the execution of the program can progress. Below is an example where it tests the contents of a text box called txtName. If it is empty then a message box will appear to prompt the user.
​
IF txtName.Text ="" THEN
Msgbox = " You must enter your Name"
END IF
​
​
RANGE CHECKING
​
Range checking investigates the limits of values entered. This becomes importanat if you need the user to enter a number between 1 and 10. See the example below:
​
BEGIN
'This program reads in a guess between 1 and 10 and tests to see if it is correct.
​
Answer = RandomNumber.Next(0, 10)
​
Guess = txtGuess.Text
​
If Guess < 1 and > 10 then
Msgbox = " You must enter a number between 1 and 10."
Else
​
IF Guess <> Answer then
Msgbox = "You are wrong"
Else
Msgbox = "You are correct"
End IF
END IF
END
​
TYPE CHECKING
​
Sometimes a user can enter the wrong data type. In Visual Basic the best test for data type is to check if the input is numerical. See the example below.
​
​
IF txtAge.Text = IsNumeric(IntAge) THEN
Msgbox =" Thank you for entering your age"
ELSE
Msgbox = "You can not enter text in this field, please enter your age as a number"
END IF
​
Logic
Programming requirements for logic layer:
-
use program control structures: selection, iteration and sequencing
​
​
SELECTION
​
​
Selection control struction allows the program to make decisions based on the data input or the selections made by the user. Commonly this if called an IF Statement. IF Statments can control the entire structure of a program. CASE Statements allow for selections to be made but are not used to control the structure of a program.
​
The example IF Statement below makes decisions based on the input of the user. If the user types a value that is the same as IntAnswer then it will return a message box prompting the user that their "guess" is correct. If guess is not correct it will check to see if the guess is higher or lower than IntAnswer.
​
If IntGuess >= 0 And IntGuess <= IntHighNumber Then
If IntGuess = IntAnswer Then
​
MsgBox("CORRECT")
Me.Close()
ElseIf IntGuess > IntAnswer Then
MsgBox("Lower")
Me.Close()
Else IntGuess < IntAnswer Then
MsgBox("Higher")
Me.Close()
End If
​
​
The example CASE Statement below allows for the user make a selection and set up parameters. Here the user makes a selection from a ComboBox "cbxLevel" which sets the difficulty of the guessing game. If the first Option is selected, Case 0 sets the Number of Guesses allowed to 10, the range of the possible correct answers to 0 - 10 and sets the correct answer. It then prompts the user.
​
Select Case cbxLevel.SelectedIndex
Case 0
IntNumber = 10
IntAnswer = RandomNumber.Next(0, 10)
IntHighNumber = 10
lblGameRules.Text = "Level 1:You have 10 guesses, the answer is between 0 and 10"
Case 1
IntNumber = 5
IntAnswer = RandomNumber.Next(0, 50)
IntHighNumber = 50
lblGameRules.Text = "Level 2: You have 5 guesses, the answer is between 0 and 50 - GOODLUCK!"
Case 2
IntNumber = 3
IntAnswer = RandomNumber.Next(0, 100)
IntHighNumber = 100
lblGameRules.Text = "Level 3: You have 3 guesses, the answer is between 0 and 100 -FINGERS CROSSED!"
End Select
​
ITERATION
​
​
Iteration means repetition. Computers are much better at mindless repetition than humans. Initially there are TWO types of interation or LOOP
1. Counted
2. Uncounted.
​
A COUNTED LOOP uses a counter variable to control how many repetitions occur. The example below asks the user three times to "Take a Guess". A FOR loop does not require a variable for the counter to be declared in Visual Basic. The counter, however, can be used as a varibale inside the FOR loop.
​
​​
​
For Counter= 1 to 3
​
Guess = InputBox("Take a guess")
Msgbox=("This is guess number " Counter)
NEXT
​
​
The FOR loops above is preset to the number of times it can repeat.
​
Below is a PRE-TEST DO loop. The loop will only run UNTIL the condition is met at the beginning.
​
​
Dim Counter As Integer
​
Counter = 1
​
Do Until Counter = 3
Guess = InputBox("Take a guess")
Msgbox=("This is guess number " Counter)
​
​
Counter = Counter + 1
​
LOOP
​
​
​
Below is a POST-TEST DO loop. The loop will only run UNTIL the condition is met at the end. This means the counter needs to be set to zero to start.
​
​
Dim Counter As Integer
​
Counter = 0
​
Do
Guess = InputBox("Take a guess")
Msgbox=("This is guess number " Counter)
​
​
Counter = Counter + 1
​
Until Counter = 3
​
​
​
AN UNCOUNTED LOOP uses other parameters to control the number of repetitions. These can be POST or PRE TEST loops. Continuing with the Guessing Game example, the first code sample below sets the Guess to 0 before the loop. This is a PRE TEST Loop for the WHILE loop to start both Guess and Answer need to contain data.
​
Guess = 0
WHILE (Guess <> Answer)
​​
Guess = InputBox("Take a guess")
​
END WHILE
​
​
​
FOR EACH is a loop that can be used to control data in an Array. Below is an example where an Array filled with student percentage scores is accessed one array item at a time. Each item is tested to be between 50 and 100 or belowo 50. This identifies the "passes" in the list. It returns the list of passed results.
​
Dim numberSequence() As Integer = {63, 43, 66, 57, 67, 98, 55, 33, 37, 79}
For Each number As Integer In numberSequence
If number >= 50 And number <= 100 Then
Console.WriteLine(number.ToString + " is a PASS")
Continue For
End If
Next
​
SEQUENCING
​
Controlling your program structure is what designing an algorithm is all about, ensuring each direction is in the correct order to get the right output. Visual Basic has it's own structure you need to be aware of. All executable code must be inside a procedure. Each procedure, in turn, is declared within a class, a structure, or a module that is referred to as the containing class, structure, or module.
​
Import Statments: You may need to import data, names or code from outside of the project.
Examples
​
Imports System.IO (allows the connection to an external text file)
Imports System.XML (allows the connection to an external XML file)
​
Class Statements: Defines a Class which contains variable definitions, object properties, events and procedures.
​
Public Class FrmGuessing Game
​
End Class
​
​
Module Statements: Modules are similar to Classes but the data they hold can only be used once. Classes are more flexible with many instances available using objects and can implement interfaces. Modules do not use objects and have only one instance of the data. They can not run more than once in an instance.
​
MODULE modAddition
​
Public Sub AddNumbers (ByVal IntNumber1 As Integer, ByVal IntNumber2 AS Integer)
​
Dim IntAnswer As Integer
​
IntAnswer = IntNumber1 + IntNumber2
MsgBox = IntAnswer
​
End Sub
​
END MODULE
​
Only code inside of the modAddition Module can see a Private Sub. If you want the Sub or Function to be available to all the code in your project, the Sub needs to be Public.
Private Sub Button1_Click (sender As Object, e As eventsArgs) _ Handles Button1.Click
​
Dim IntNumber1 as Integer
Dim IntNumber2 as Integer
IntNumber1 ← txtNumber1.Text
IntNumber2 ← txtNumber2.Text
​
Call AddNumbers (IntNumber1, IntNumber2)
​
End Sub
​
​
Function Statements: A Function procedure is a series of Visual Basic mathematical procedures enclosed by “Function” and “End Function” and returns a value to the code that called up the function.
​
​Public Function DivideByThree (ByVal IntAnswer as Integer)
​
Dim DblThird As Double
DblThird = IntAnswer / 3
return DblThird
END Function
​
Below is an example where the Function and the SubRoutine are called up in an Event (Button_Click)
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
​
Sub Statements: A sub Statement declares the name, parameters, and code that define a Sub procedure. You use a Sub procedure when you don't want to return a value to the calling code. Use a Function procedure when you want to return a value. If you have an object that triggers an event such as a button you would define the procedures as a Sub where global variables can be passed in and accessed.
​
​
Declare Statments: All variables need to be declared before they can be assigned values, used in functions or modified. In a declaration statement you use "Dim" which is a shortened version of the term Dimension.
​
Dim Name As String = txtName.Text
Dim Age As Integer = Val(txtAge.Text)
Dim FinalResullts() As Double
​
​
Operator Statements: Declares the operator symbol, operands, and code that define an operator procedure on a class or structure.
​
IsFalse, IsTrue IsNumeral, NOT, AND, LIKE, MOD, OR, XOR, +, -, *, /, >,<, <>, >>, <<, =<, >=, &
​
Property Statements: List of attributes that apply to an Object or Get or Set procedure. The most common property we control in the code is .Text. The example below shows the data "Bill" will be applied to the TEXT property of the object txtName.
​
txtName.Text = "Bill"
​
Event Statments: Declares a user-defined event. To handle an event, you must associate it with an event handler subroutine using either the Handles or AddHandler statement.
​
Logic
Programming requirements for logic layer:
-
use modularisation and code optimisation
​
It is important that your code runs quickly and effectively. It will be necessary to group your code into modules and use functions to be used repeatedly rather than writing the calculations in each instance where necessary. Some control structures are best suited for certain applications and it will be up to you to decide how best to use them. Microsoft has a list of Optiisation suggestions for Visual basic here.
​
​
Logic
Programming requirements for logic layer:
-
use objects, methods and event-driven programming functions.
​
Object-Oriented Programming (OOP) is a method of software design and construction. It is the next logical progression, after structured programming, to improve your code reusability and maintainability. Put another way, OOP is a method of designing individual software components (classes) with associated behaviors (methods) and data limitations (properties), and that helps you piece these components together to create a complete application.
​
Objects are used in Visual Basic to build interfaces (Forms, Buttons, Textboxs, ComboBoxes etc.) as well as Functions and Modules.
​
A method is an action that can be performed by an object. In Visual Basic .NET, methods are defined as Subs and Functions created in a Class.
​
An event is a signal that informs an application that something important has occurred. For example, when a user clicks a control on a form, the form can raise a Click event and call a procedure that handles the event. Events also allow separate tasks to communicate. Say, for example, that your application performs a sort task separately from the main application. If a user cancels the sort, your application can send a cancel event instructing the sort process to stop.
​
​
Data Source
Programming requirements for data source layer:
-
design, construct and use external storage and access technologies
-
retrieve data from external sources.
​
There may well be more than three ways of accessing external data storage but here I will present:
-
Access Tables
​
​
TEXT FILES
Text Files are the easiest way of accessing data and saving data, but you can only have simple lists. Befor you declare your Class you need to Import a Text file connector:
​
Imports System IO
​
If I had a list of names I could enter them into a txt file and save it as "Names.txt" into the Bin folder of my VB Application. In the Code I would declare and import Names.txt into a Listbox. See example below:
​
Dim Names() As String = IO.File.ReadAllLines("Names.txt")
Listbox1.Items.AddRange(Names)
​
You can also use StreamReader to read in and make changes to the text file. For more detailsed examples see this sheet. Ideally if you have simple 1D Arrays in your solution a text file may suffice.
​
XML FILES
​
XML is a text form of a spreadsheet. They allow for RECORDS. Excel easily exports any spreadsheet as an XML file and it is easy to create and read in XML files to Visual Basic. If you are using small databases of records and searching by FIELD you may like to use XML.
​
XML is similar to HTML using tags to Identify records and fields. An example below shows two records:
​
<?XML version = "1.0"?>
​
<Customers = "Customer Details">
​
<Customer Name = Jack Johnston">
<membership>TRUE</membership>
<contact> 0467543264</contact>
<DOB>16/4/1989</DOB>
​
<Customer Name = Brendan Smith">
<membership>TRUE</membership>
<contact> 0434749062</contact>
<DOB>22/7/1976</DOB>
​
</Customers>
​
For more detailsed examples see this sheet.
​
​
​
ACCESS TABLES
If you create tables in Access they can be connected to your VB program. The documentbelow outlines how you can create a Login process that checks the user's password from an Access database.
​
​
​
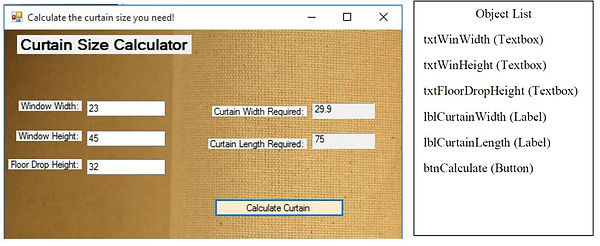
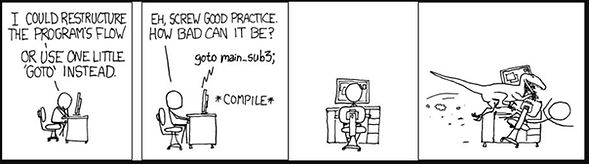