VCE Software Development Units 3 & 4
Unit 3 Outcome ONE
Outcome One Notes
Data and Information
Information Systems are any organisation system that sorts, finds, retrieves displays information. Information is processed data. The diagram below (Fig1) illustrates the input of data to the process and then exported as information. An example might be your mobile phone as an information system for phone contacts. You input the data - given names, family names and mobile numbers and the system places the data into the memory which can then be sorted, searched and used. Data are organised, sorted and formatted into information such as spreadsheets, magazine layouts, websites, or reports to name a few. In digital systesm we mainly focus on text, numbers and images in our databases, software development and web development.
Data comes in five digital types:
-
text
-
numbers
-
images
-
sound
-
video
All of these data types are composed of fundamentally the same thing. Numbers! Digitising any data is the process of converting it into numbers.
BINARY
Digital machines are build on circuitry that allows for the flow of electricity. A digital machine can detect if a circuit is ON (where there is electricity flowing through the circuit) or OFF (no electricity) . This is the only way these machines can "understand" the data that is read in. So we devised a simple code to bridge the divide between humans and machines. If the circuit is OFF we will call it a zero. Computer scientists use Ø to distinguishi it from the letter O. If the circuit is ON we will call it 1. Thus the divide between machines and humanity is made.
Humans have a number system based on 10 symbols (Ø, 1, 2, 3, 4, 5, 6, 7, 8, 9) which is called the decimal system. All values can be created with these ten symbols. It is suggested the reason we chose ten symbols was due to having ten fingers. Digital machines do not have the capacity to understand anything beyond ON and OFF, so we have to construct a number system around the two "fingers" they have: Ø and 1. Since there are only two symbols we call this number system Binary (bi means 2).
Now we have to represent all the values and all the other symbols we use in text. We call each Ø and each 1 a bit and we have to combine them to create unique identifiers for each symbol. We group eight bits together to represent all the ASCII symbols (all the letters, numbers and symbols input by a keyboard). This group of eight bits is called a byte.
MEMORY
One bit = Ø
One bit = 1
One Byte = 8 bits. Example Ø Ø 11Ø 1Ø 1
a = Ø11ØØØØ1
b= Ø11ØØØ1Ø
c = Ø11ØØØ11
! = ØØ1ØØØØ1
Bytes are too small to measure memory, as we usually store hundreds of characters in a basic text file. Binary is based on the number 2, so we if we set a kilobyte to: 2 to the power of 10 we would have 1024 bytes in a kilobyte.
Here is the size of common units of measurement of digital storage:
1024 byte = 1 Kb
1024 Kb = 1 Mb
1024 Mb = 1 Gb
1024 Gb = 1 Tb
1024 Tb = 1 Pb
DATA TYPES
It is important to be aware of common data types used in software development and their size and range. Microsoft have a full list of all data types here. The Key Data Types you need to know are:
-
Boolean
-
Character
-
Floating Point (Decimal Numbers)
-
Integer (Whole Numbers)
-
String (Text of any symbols)
VARIABLES
Variables are temporary data holding-spaces used in software. These are a crucial aspect of reading in data, processing it and displaying the output.
Here is a basic example: A simple calculator that reads in two numbers, adds them and displays the answer.
A variable would hold the value of each of the two numbers typed in by the user and then
another variable would hold the answer.
This is an Algorithm (a plan for a solution) for thea calculator using variables.
START
Number1 ← Integer
Number2 ← Integer
Answer ← Integer
Read in Number1
Read in Number2
Answer ← Number1 + Number2
Display Answer
END
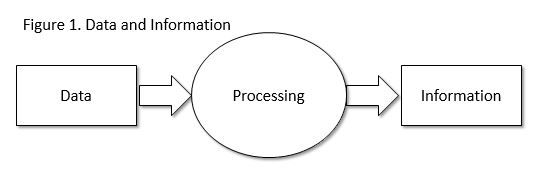
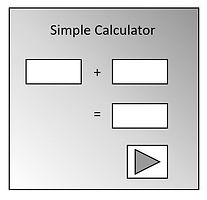
Records are made up of fields. The fields in the example above are: ID Number, Given name, Family Name, Mobile Number, Date of Birth and Address. The records can be sorted and searched by fields so that similiar records can be identified. For example all records with Date of Birth data lower than 1/1/1999 will return all records of people who are over the age of 18 years. Records are most easily managed when unique identifiers are included, such as ID Number. This ensures all records are unique and we can easily distinguish the possible multiple Robert Smiths recorded in the file.
In an algorithm we can place a new record like this:
START
CustomerContacts.IDNumber ← 3 (Data Type = Integer)
CustomerContacts.GivenName ← Lady (Data Type = String)
CustomerContacts.familyName ← Gaga (Data Type = String)
CustomerContacts.MobilePhone ← 0488 239 765 (Data Type = String)
CustomerContacts.DateofBirth ← 28/3/89 (Data Type = Date/Time)
CustomerContacts.Address ← 18 Hollywood Boulevard (Data Type = String)
END


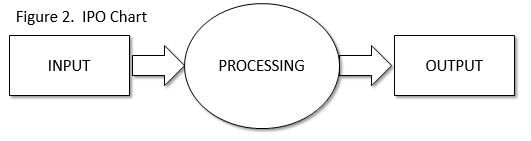
For the Simple Calculator example
INPUT = Number 1 and Number2
OUTPUT = Answer
PROCESS = Number1 + Number2 = Answer
This is agreat place to start when designing a complex system solution. Identifying the data that goes in and what comes out is very helpful!
DATA DICTIONARY
Now that we know what data needs to handled we need to design the variables to handle it. Creating a Data Dictionary is an important aspect part of the Design Stage of developing a solution. It includes the names of the variables, the data types of the variables, the size of the data held and a description of the data. Returning to our Simple Calculator again here is a Data Dictionary.
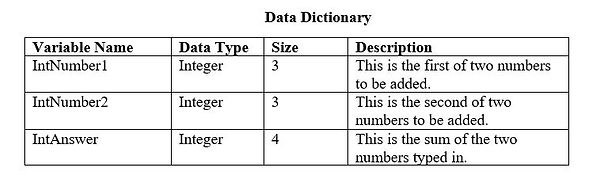
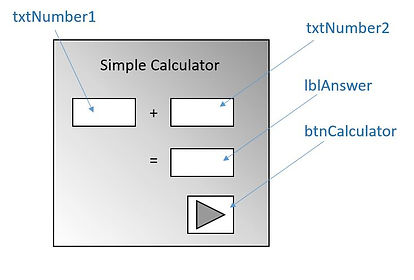
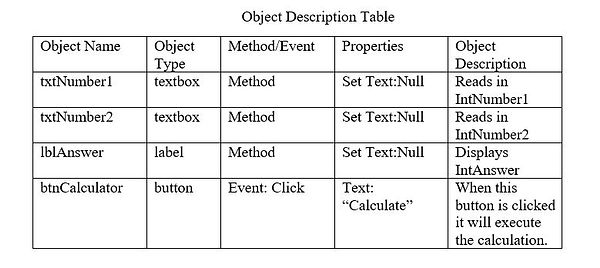
Now that we have identified our objects, we can create an Object Description Table. This is way of formally identifying the name, type, purpose and properties.You can see in the Object Description Table above that there is a column titled: Method/Event. This identifies how the objects are affected by things that happen (events) during the execution of the program or if the properties of the object are changed (methods). For example: lblAnswer is a label that will have its "text" property changed in the execution of the program, while the button btnCalculator triggers the program execution when the event_Click occurs.
MOCK UP - DESIGN LAYOUT
A Mock Up or Design Layout is a plan that identifies what the GUI will look like.
The illustration on the right shows a rough hand drawn design for the interface of
the simple Calculator. It identifies each of thee objects and their purpose as
well as design elelments.
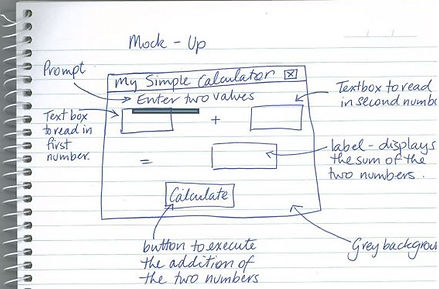
PSEUDOCODE
Once you have decided on the GUI, the objects and variables required to solve your programming problem, it is time to define the events that take place when executed. Rather than going directly to Visual Studio and typing up your code, it is essential that you plan your structure in an algorithm using pseudocode. Pseudocode is natural language that is easy to read but follows the strucutre of a programming lanaguge.
The Features of Pseudocode
-
Every algorithm must begin and end with START and STOP or BEGIN and END.
-
When assigning data to a variable use ←. Example: IntNumber ← 5
-
Show each calculation required Example: IntAnswer ← IntNumber1 + IntNumber2
-
Displaying data as output Example: lblAnswer.Text ← IntAnswer or Display IntAnswer
-
Decision Control Structures: Example: IF condition THEN Action1 ELSE Action2 ENDIF
-
Case Control Structures: Example: Case where condition, CASE1 Action1 CASE2 Action2 END CASE
-
Repetition Control Structures: Example: FORcounter ← first value to last value END FOR
-
Repetition Control Structures: Example: REPEAT actions UNTIL condition
-
Repetition Control Structures: Example: WHILE condition DO actions END WHILE
-
Use indentation and spaces to illustrate how control structures are incorporated
Below is an Algorithm written in Pseudocode that allows the user three attempts at getting their username and password correct. The program checks the password is correct at each attempt and prompts the user if they are wrong and to try again until they have enter the correct combination.
START
strUsername ← BillBurr123
strPassword ← Summer1965
Read in strUsernameEntered
Read in strPassswordEntered
Counter ← 0
WHILE strUsername <> strUsernameEntered AND strPassword <> strPassswordEntered AND Counter < 3
Display Prompt "Your Username and Password are incorrect please try again!"
Read in strUsernameEntered
Read in strPassswordEntered
Counter ← Counter +1
END WHILE
Display Prompt "Your Username and Password are correct!"
END
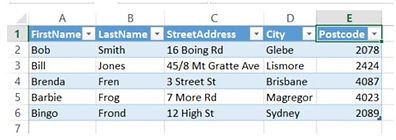
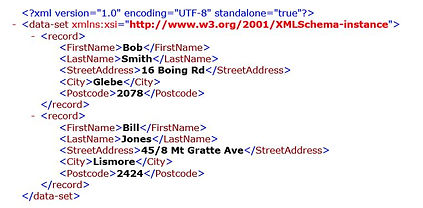
Download these examples:
-
To create your own XML from Visual Basic access this example: Excel to XML
-
For more examples of file access: Visual Basic and accessing external files
-
In some cases Microsoft Access is required to store more complex record structures: Access and Visual Basic
Validation
The interface between machines and humans is frought with opportunities for data loss, mistakes and possible nefarious intentions.
Validation is the process of checking the input of data. Manual validation includes spell checking, proof reading and fact checking. These are processes the user can conduct before entering data into a system. As a software engineer, it is important to anticipate human error when reading in data to your program.
Common validation techniques include:
-
Existence Checking
-
Type Checking
-
Range Checking
Existance Checking is a validation method that checks if data is present in a variable or object. This can be an important aspect of your interface design. If the user has not included an important data element existance checking will detect it and promp the user to enter the missing data. For example: in a pizza ordering app, if the user has not selected a pizza type, it is impossible to process an order, so a message window would appear to prompt te user to make a pizza type choice.
Type Checking is a validation method that checks the data type of the data entered by the user. This is vital to detect typing errors. If a "Q" is typed into the input object for "Number of Pizza's", type checking will detect that the data type is not numerical and return a prompt to the user to enter a "Number" to indicate how many pizzas they want to order.
Range Checking is a validation method that checks if a value sits between two limits. A common use of range checking would be on the input of "date of birth". To ensure those logging in to an app are over 16, the range check could test for dates after 1/1/2001. Similarly, dates before 1901 would also be excluded due to the unlikelyhood that users would be over the age of 100.
Unit 3: Software Development
In Software development Units 3 and 4 students focus on the application of a problem-solving methodology and
underlying skills to create purpose-designed solutions using a programming language. In Unit 3 students develop
a detailed understanding of the analysis, design and development stages of the problem-solving methodology
and use a programming language to create working software modules.
In Area of Study 1 students respond to given software designs and develop a set of working modules through
the use of a programming language. Students examine a range of software design representations and interpret
these when applying specific functions of a programming language to create working modules. In Area of Study 2
students analyse a need or opportunity, plan and design a solution and develop computational, design and systems
thinking skills. This forms the first part of a project that is completed in Unit 4.
Software tools
The following table indicates the software tools that students are required to both study and use in this unit.
-
Area of Study 1 An appropriate programming language
-
Area of Study 2 Unified modelling language to create use cases
Area of Study 1
Programming practice
In this area of study students focus on the design and development stages of the problem-solving methodology
and computational thinking skills. Students examine the features and purposes of different design tools so they can
accurately interpret the requirements for working software modules. Students interpret given designs and create
working modules using a programming language, undertaking the problem-solving activities of coding, testing
and documenting (development stage). Students use a programming language that meets the programming
requirements published annually by the VCAA in the VCAA Bulletin. SKC will be using Visual Basic.
The working modules do not have to be complete solutions and can focus on limited features of the programming
language; however, students are expected to fully develop the working modules in accordance with the given
designs. Each module should allow the testing of the program logic in readiness for creating a complete solution in
Unit 4. Testing techniques are applied to ensure modules operate as intended and students learn to write internal
documentation in the code that they develop.
Outcome 1
On completion of this unit the student should be able to interpret designs and apply a range of functions and
techniques using a programming language to develop working modules. To achieve this outcome the student will draw on key knowledge and key skills outlined in Area of Study 1.
Key knowledge
Data and information
-
characteristics of data types
-
types of data structures, including one-dimensional arrays (single data type, integer index) and records (varying data types, field index)
Approaches to problem solving
-
methods of representing designs, including data dictionaries, object descriptions, mock-ups and pseudocode
-
formatting and structural characteristics of input and output, including XML file formats
-
a programming language as a method for developing working modules that meet specific needs
-
processing features of a programming language, including instructions, procedures, methods, functions andcontrol structures
-
techniques for linear and binary searching
-
techniques for checking that modules meet design specifications, including trace tables and test data
-
purposes and characteristics of internal documentation, including comments and meaningful names.
Key skills
• interpret designs to develop working modules that meet these requirements
• use a range of data types and structures
• use appropriate processing features of a programming language
• select and use appropriate techniques to test the functionality of modules
• document the functioning of modules through the use of internal documentation.
TEXT BOOK: Software Development: Core techniques and Principles 3rd Edition Adrian Janson
Unit 3 Outcome 1 Area of Study: Programming Practice.
-
Chapter 5. The Art of Design
-
Chapter 6. The structure of a programming language
-
Chapter 7. Data Structures and Data Manipulation
-
Chapter 8. Of input and output
-
Chapter 9. Testing and Evaluating
An ARRAY is a very useful data structure for large amounts of data that needs to be sorted or searched. Arrays can only hold one type of data type. Each element has an indexed address and this allows arrays to be easily manipulated. In the example below you can see a series of integers, unsorted. The row above the top identified each element's address from zero to nine. This array has 10 elements but is identified as Array[9]. Remember computers start counting at zero!
We can pull the data elements out of the array using their location, for example:
Representing Design
IPO CHART
We need to use tools to plan our software solutions. The most basic is tool is the IPO chart. This chart identifies the input and output variables and the processes inbetween.
The Data Dictionary above shows the correctly named variables, their data types and descriptions. The size "3" indicates the largest number that can be entered is 999. If we changed the size to "2" the largest number we could enter would be 99. It indicates the number of characters. We needed to put "4" as the size for IntAnswer because the largest possible value it may encounter si 999 + 999 = 1998. It is also possilbe to identify the Scope of the variable - is it limited to a subroutine, making it "LOCAL" or is it accessible from anyware in the program - "GLOBAL".
OBJECT DESCRIPTION TABLE
When designing your interface, especially in Visual Basic, you need to identify objects that will handle your variables, and in turn, your data. It is important to use CamelCase and Hungarian Notation in your naming of objects as well. You can see by the Graphical User Interface (GUI) below that the two INPUT objects are text boxes (txtNumber1 and txtNumber2). The OUTPUT will be displayed in a label (lblCalculator). The entire program will be executed when the button object (btnCalculator) is clicked.
Formating and Structural Characteristics of Input and Output
So far we have only discussed software solutions where the user enters the data via a keyboard and mouse. This is not always the most efficient method especially when we have large amounts of complex data. Sometimes data is collected from CCTV capture such as car registration plates moving through an intersection. Collecting data out in the field may require locations to be input which could be collected via a GPS device.
When dealing with large amounts of data it is important that the data is structured in such a way that it can be accessed, sorted, searched, saved and retrieved. Software solutions such as our Simple Calculator do not save data for use after the program has been turned off.To create effective, robust software solutions these programs need to save data perminantly so it can be accessed again after the software has been shut down. This requires files that can hold the data.
A fundamental approach is to use a file such as a text file that you can edit in Notepad. This is a great approach is you are making a simple application where the client needs to update prices. The link below illustrates how a simple list in a text file can be accessed from a Visual Basic program. The example shows that the list is easy to update for a client. The list is read into a pull-down menu: Accessing Text in Visual Basic.
If you need a more structured approach such as the use of a spreadsheet you might need to upgrade from a basic text file to an XML file. when you have data structures such as records, it is best to be working with fields. XML uses tags in much the same way HTML is structured. The table below shows a 5 records. It is described and controlled by the XML file displayed below.
Programming Languages
Programming Languages are coded instructions that both a human ana machine can understand. There are two main types of programming languages: interpreted and compiled. An interpreted language requires software to interpret and run the code. These lanaguages rely on the browser software to interpret and run the code in their window.A common example of interpreted languages are those that run inside browsers on websites such as:
-
PHP
-
Excel
-
XML
-
HTML
-
JavaScript
-
Perl
Compiled Languages are converted into machine code and can run independently of other software. The code can be compiled in an Integerated Development Environment (IDE) or through a text editor. The create a stand-alone software application. Languages include:
-
Python
-
Visual Basic
-
C++
-
C#
-
Java
-
Pascal
Visual Basic is a compiled Object Oriented Programming (OOP) language. It enables the user to design a GUI easily with a point-a-click process in the IDE. The GUI is designed by arranging input, output and processing objects on a window or form. Each object is named and its properties editied according to its association witha variable.
PROCEDURES, SUBROUTINES, EVENTS AND MODULES
In Visual Basic there is a hierarchy of coding segmentation. A complete Visual Basic application is called a PROJECT the default name is WindowsApplication1. Within a project there may be many code files called MODULES. These maybe called something like Form1.vb.
Once a Module has been created we write the PROCEDURES as code. In VB there are two types of procedures; 1 FUNCTIONS and 2. SUBROUTINES.
Functions perform tasks that return a value.
Example:
IntAnswer = IntNumber1 + IntNumber2
Subroutines perform tasks but may not return a value.
MODULE modAddition
Public Sub AddNumbers (ByVal IntNumber1 As Integer, ByVal IntNumber2 AS Integer)
Dim IntAnswer As Integer
IntAnswer ← IntNumber1 + IntNumber2
MsgBox ← IntAnswer
End Sub
END MODULE
Only code inside of the modAddition Module can see a Private Sub. If you want the Sub or Function to be available to all the code in your project, the Sub needs to be Public.
Private Sub Button1_Click (sender As Object, e As eventsArgs) _ Handles Button1.Click
Dim IntNumber1 as Integer
Dim IntNumber2 as Integer
IntNumber1 ← txtNumber1.Text
IntNumber2 ← txtNumber2.Text
Call AddNumbers (IntNumber1, IntNumber2)
End Sub
A Function procedure is a series of Visual Basic mathematical procedures enclosed by “Function” and “End Function” and returns a value to the code that called up the function.
Public Function DivideByThree (ByVal IntAnswer as Integer)
Dim DblThird As Double
DblThird ← IntAnswer / 3
return DblThird
END IF
Below is an example where the Function and the SubRoutine are called up in an Event (Button_Click)
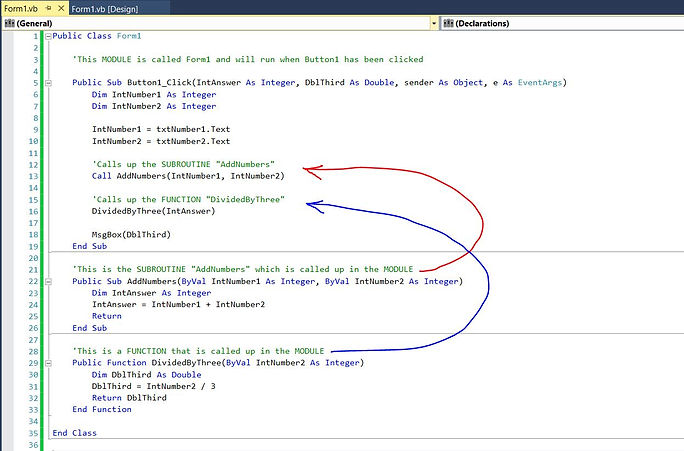
CONTROL STRUCTURES
To control the order in which your functions and procedures are executed, some structure needs to be put in place.
There are three main control structures:
-
Sequence
-
Selection
-
Iteration
Sequence is where one process follows another in the correct order.
Example
START
Read In Number1 (Procedure 1)
Read In Number2 (Procedure 2)
Answer ← Number1 + Number 2 (Procedure 3)
Display Answer (Procedure 4)
END
Decision control structures determine the direction of the program based on condition statements.
Example
START
Read In Number1
Read In Number2
Answer ← Number1 + Number 2
IF Answer Mod 2 > 0 THEN (IF condition is TRUE it will do Procedure 1)
Display "The Answer is EVEN!" (Procedure 1)
ELSE (IF codition is FALSE it will dow Procedure 2)
Display "The Answer is ODD!" (Procedure 1)
END IF
END
ITERATION is a control structure that repeats a set of procedures in a loop. These loops can be controlled by counting the number of times the loop completes or by testing conditions.
Counted Iteration Example: This algorithm will read in three guesses via an input box.
START
Writeline: Guess the Number! You get three guesses!
For Counter 1 to 3 Do
Guess ← Input Box( Take a guess )
End For
END
Pre-test Loop Example: This algorithm shows the loop commences if the condition (the guess is wrong) is true.
START
Writeline: Guess the Number! Keep guessing till you get it right!
Guess ← Input Box( Take a guess )
While Guess <> Answer
Guess ← Input Box( Take a guess )
End For
END
Post-Test Loop Example: This algorithm tests the outcome of the first loop and keeps looping until the condition is met.
START
Writeline: Guess the Number! Keep guessing till you get it right!
REPEAT
Guess ← Input Box( Take a guess )
UNTIL Guess = Answer
END
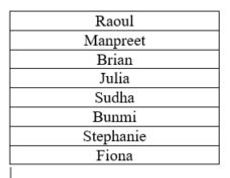
LINEAR SEARCH
If we were looking for "Bunmi" manually in our list of names, we might start at the top and run our finger down the list to check each element. Linear Search does exactly that. It begins at the beginning of the array and uses a loop to check each elelment until it is found. Below is an algorithm to serach fro Bunmi in the list above.
START
Counter ← 0
Found ← False
While Found = False AND Not EOF DO
If Names (Counter) <> Bunmi THEN
Counter ← Counter + 1
ELSE
Found ← TRUE
END IF
END WHILE
END
This type of searching approach is fine for small lists, but if you have a telephone directory of data to search this is very inefficient.
BINARY SEARCH
Clearly we need a better method than a Linear Search for larger files, and that is where Binary search is
useful. Unfortunaley the list must be SORTED first to conduct a binary search.
You can see our list Names(7) on the right has been put into alphabetical order making it easier to serach.
Names (0) = Brian
Names (1) = Bunmi
Names (2) = Fiona
Names (3) = Julia
Names (4) = Manpreet
Names (5) = Raoul
Names (6) = Stephanie
Names (7) = Sudha
First we define the lowest, highest and middle elements of the list.
LOWEST element ← Names (0) = Brian
MIDDLE element ← Names (4) = Manpreet
HIGHEST element ← Names (7) = Sudha
Now we check the name we are searching for "Bunmi" against the MIDDLE element.
Is Bunmi > or < Manpreet in the alphabet? Bunmi is < (or before) Manpreet. So we now redefine our lowest, middle and highest. Because Bumin is before Manpreet we make the old MIDDLE the new HIGHEST and then find a new MIDDLE.
LOWEST element ← Names (0) = Brian
MIDDLE element ← Names (2) = Fiona
HIGHEST element ← Names (4) = Manpreet
Again we test to see if Bunmi is > or < our new MIDDLE element Fiona. Since Bunmi is before set the MIDDLE to be the new HIGHEST once again.
LOWEST element ← Names (0) = Brian
MIDDLE element ←Names (1) = Bunmi
HIGHESTelement ← Names (2) = Fiona
Now once again we check our MIDDLE element and we have found our name "Bunmi".
COMPARISION OF LINEAR AND BINARY SEARCHES
It is clear that a Linear search needs to potentially conduct as many operations as there elements in the list being searched. Binary was the 6th element in the unsorted list so at least 6 operations would have been required to find that name.
However, with the Binary search only 3 operations found the same element. How many operations would take to find Stephanie?
Operation 1
LOWEST element ← Names (0) = Brian
MIDDLE element ← Names (4) = Manpreet
HIGHEST element ← Names (7) = Sudha
Stephanie > Manpreet so MIDDLE is the new LOWEST
Operation 2
LOWEST element ← Names (4) = Manpreet
MIDDLE element ← Names (6) = Stephanie
HIGHEST element ← Names (7) = Sudha
Found in 2 Operations.
Testing Solutions
While developing a solution you need to test it to see if what you have coded works. Sometimes it is easy to see if it works especially if there are syntax errors. The code will not execute if there is a syntax error. If the output of your code is wrong you may have a logic error and this can be tricky to find in larger more complex programs.
TEST DATA
First we need to develop a table of Test Data. The test data needs to include the kind of input that would be expected of the user. For example let's look at this guessing game program:
START
Writeline: Guess the Number! The number is between 1 and 10!
For Counter 1 to 3 Do
Guess ← Input Box( Take a guess )
End For
END
The expected input would be "1, 2, 3, 4, 5, 6, 7, 8. 9 10". But would would happen if you entered "127", or "eleven", or "*^$&(?@!" ? Programmers need to build solutions that can handle any input . For example: the correct response for INPUT = 127 would be "You must enter a number between 1 and 10". The correct response to INPUT = eleven or INPUT = *^$&(?@! would be "You must enter a number not other characters".
When creating a TEST TABLE you must include:
-
Valid Input (Example: 1, 2, 3, 4, 5, 6, 7, 8. 9 10)
-
InValid Input (Example: eleven or 127)
-
Unexpected Input (Example: (*&*%#%$)
TRACE TABLES
Trace Tables are designed to find logic errors when valid data is entered but incorrect output data emerges. We list all the variables across the top and enter Valid input and follow the program line by line entering the changes to the values in the variables as we go.
Lets look at a simple example:. Below is a program that sets the Answer to the guessing game at 6. All three guesses will be read in and placed into an array called Guess (2) . The first FOR loop reads in the guesses into each array element. The second FOR loop checks the array for a correct answer and assigns points based on whether it was the first, second or third guess.
START
Declare Guess (2) ← Integer
Declare Answer ← 6
Declare Points ← Integer
Writeline: Guess the Number between 1 and 10!
Writeline: You get three guesses!
For Counter 0 to 2 Do
Guess(Counter) ← Input Box( Take a guess )
End For
Writeline: You have entered your 3 Guesses let's see if one is correct!
For Counter 0 to 2 Do
IF Guess(Counter) = Answer THEN
IF Counter = 0 THEN
Points ← 10
ELSEIF Counter = 1 THEN
Points ← 5
ELSEIF Counter = 2 THEN
Points ← 1
ENDIF
Writeline: You got it right! You have (Points) number of points!
ELSE
Writeline: You you didn't guess the answer!
END IF
END FOR
END
First we need TEST TABLE but since we have not added any validation to ensure invalid or unexpected data is edited let's just check valid input to find any logic errors. Each set of data tests a differnt aspect of the program the first set of data tests a correct answer
for Guess(1), the second set of data tests no correct answer, the third set of data tests a correct answer for
Guess(0), etc.
All possible correct solutions are tested and there are two sets that test for no correct answer.
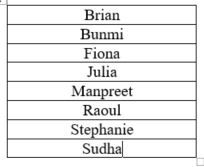
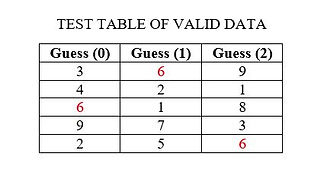
So now let's put the first set of data into a TRACE TABLE. Across the top of our trace table are all the variables used in the program and down the side identifies each operation in the code.
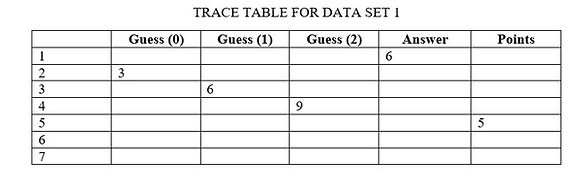
You can see the first line contains the set Answer as 6, then each of the guesses for each iteration of the FOR loop. Finally 5 points are allocated because Guess(1) contained the correct answer.
Internal Documentation
When writing software you should always mark your work clearly to indicate how it functions. There is nothing more annoying than coming back to a complex solution you have written in past, only to discover you can not remember what each variable is, or even what each section of code is doing. To avoid this, and to assist your clients in updating the code in the future, it is important to name your objects and variables according to type and purpose. Also non-executable comments can be placed throughout the code to describe the function of the code.
VARIABLE & OBJECT NAMING
If you are coding in Visual Basic the first stage is to build the GUI. You might use a text box to read in a data element such as Window Width (see the example below). To name the object it is a common naming convention to include the object TYPE (Textbox) with the object's PURPOSE (Window Width). Object names need to be an uninterrupted string without any spaces so we use CAPTIALS to break up the name. For example: txtWinWidth. This approach is called Camel Casing and makes the name easy to read. The combination of type and purpose is called Hungarian Notation.
See the example below of a Curtain Calculator. A common confusion occurs when there are two similar objects - in this case, reading in the width of the window and the calculation of the curtain width. The object names clearly distingish these two objects.
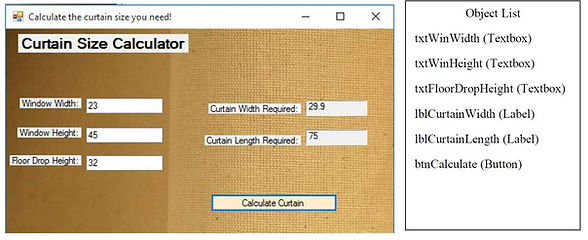
Similarly the names of your variables should contain the DATA TYPE and the PURPOSE. Continuing with the Curtain Calculator example the variable that is handled by txtWinWidth is a floating point value called fltWinWidth. If you investigate the solution below, regardless of your experience with Visual Basic, you can clearly follow what the program does.
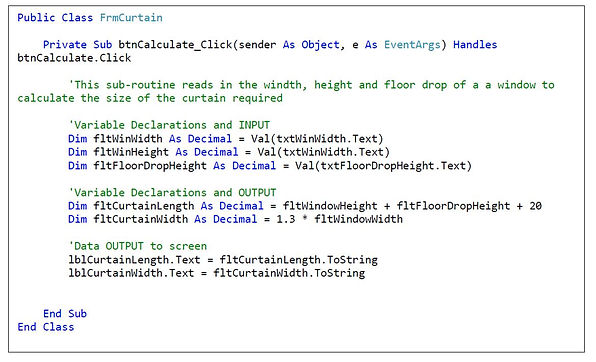
COMMENTS
Comments are not part of the executable code. They do not slow down the operation of the program and do not affect the the way the software functions. Comments do, however, make understanding the code so much easier. With fully documented code highly complex software solutions can be easily understood when you return to the program later, or if you need to hand it over to someone else for editing. In the Curtain Calculator above you can see the comments in green.
END OF U3O1
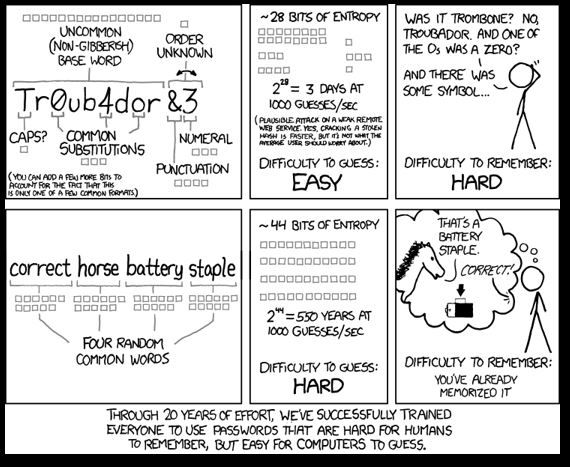